To upgrade:
bun upgrade
To install:
curl https://bun.sh/install | bash
If you have any problems upgrading
In Bun v0.1.11, web framework libraries built on Bun got faster
Benchmark: https://github.com/SaltyAom/bun-http-framework-benchmark This was run on Linux Image credit: @Kapsonfire-DE
Plugin API
Bun's runtime now supports a plugin API.
- Import and require
.svelte
,.vue
,.yaml
,.scss
,.less
and other file extensions that Bun doesn't implement a builtin loader for - Dynamically generate ESM & CJS modules
The API is loosely based on esbuild's plugin API.
This code snippet lets you import .mdx
files in Bun:
import { plugin } from "bun";
import { renderToStaticMarkup } from "react-dom/server";
// Their esbuild plugin runs in Bun (without esbuild)
import mdx from "@mdx-js/esbuild";
plugin(mdx());
// Usage
import Foo from "./bar.mdx";
console.log(renderToStaticMarkup(<Foo />));
This lets you import yaml
files:
import { plugin } from "bun";
plugin({
name: "YAML",
setup(builder) {
const { load } = require("js-yaml");
const { readFileSync } = require("fs");
// Run this function on any import that ends with .yaml or .yml
builder.onLoad({ filter: /\.(yaml|yml)$/ }, (args) => {
// Read the YAML file from disk
const text = readFileSync(args.path, "utf8");
// parse the YAML file with js-yaml
const exports = load(text);
return {
// Copy the keys and values from the parsed YAML file into the ESM module namespace object
exports,
// we're returning an object
loader: "object",
};
});
},
});
We're planning on supporting browser builds with this plugin API as well (run at transpilation time)
Reliability improvements
Node compatibility:
- feat: implement native os module by @xHyroM in https://github.com/oven-sh/bun/pull/1115
- fix buffer.copy by @zhuzilin in https://github.com/oven-sh/bun/pull/1113
- fix buffer.slice(0, 0) by @zhuzilin in https://github.com/oven-sh/bun/pull/1114
- Add buffer.indexOf, includes and lastIndexOf by @zhuzilin in https://github.com/oven-sh/bun/pull/1112
- Add native EventEmitter by @zhuzilin in https://github.com/oven-sh/bun/pull/1123
- Support emit Symbol events in EventEmitter by @zhuzilin in https://github.com/oven-sh/bun/pull/1129
- add SlowBuffer by @zhuzilin in https://github.com/oven-sh/bun/pull/1133
- update minified url polyfill by @samfundev in https://github.com/oven-sh/bun/pull/1132
- Add pad back to base64 by @zhuzilin in https://github.com/oven-sh/bun/pull/1140
- fix mkdtemp by @zhuzilin in https://github.com/oven-sh/bun/pull/1151
- Fix Buffer.isEncoding 39dc9899157d082841d79daa2f98dbecaa4efed6
- Implement
napi_add_finalizer
f023b89b732db0aff24445acbbe39c366d13118d NAPI_MODULE_INIT()
wasn't implemented correctly in Bun and that has been fixedimport assert
andimport process
did not behave as expected (assert
wasn't returning a function). This has been fixed"node:module"
'screateRequire
function wasn't requiring non-napi modules correctly
macOS event loop internals moved to a more reliable polling mechanism:
setTimeout
CPU usage drops by 50% https://github.com/oven-sh/bun/commit/c1734c6ec5ef709ee4126b3474c7bee0a377a1fa (Before: 90%, After: 33% - still more work to do here)- on macOS, bun would sometimes hang due to race conditions (unrelated to network connection) if you ran
fetch
enough times in quick succession. The race conditions have been fixed.
More:
- [bun:ffi] Fix crash with uint64_t 296fb41e920736041c6c1dec38f1d8c355a402a1
- [bun:ffi] Fix int16 / uin16 max 30992a8b051565ace57083b990d010316d56605d
- Fix
Request
andResponse
in macros e0b35b3086b00fb27f950a72a082b360a3dad891 - Fix
clearTimeout
on Linux e6a1209c53adb3056263b894d774b30ee70a3188
Performance improvements
Bun has a long-term commitment to performance. On macOS, React server-side rendering gets around 2x faster.
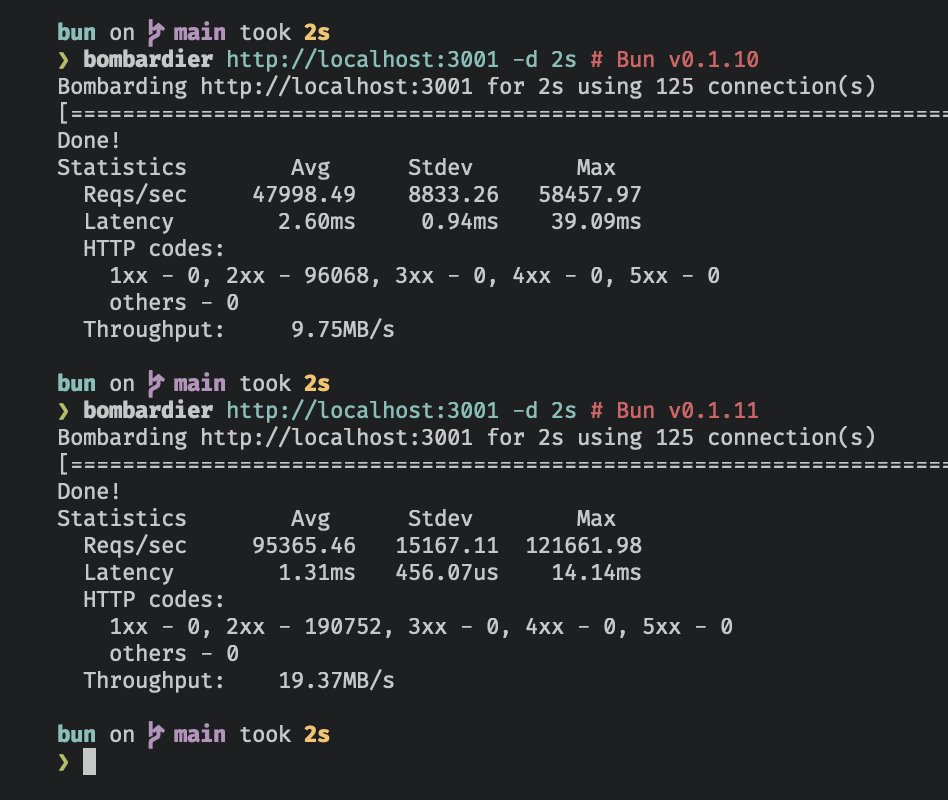
Coming up next in performance improvements: a new HTTP server implementation. Not far enough along for this release, but experiments are showing progress.
PRs
- fix(ReferenceError): expected type in getCode by @xHyroM in https://github.com/oven-sh/bun/pull/1120
- chore: fix bun-tools location in macOSx Zig instructions by @mljlynch in https://github.com/oven-sh/bun/pull/1124
- Fix clearTimeout and linux timeout by @zhuzilin in https://github.com/oven-sh/bun/pull/1138
- Add native BufferList by @zhuzilin in https://github.com/oven-sh/bun/pull/1146
- fix: broken README links by @MNThomson in https://github.com/oven-sh/bun/pull/1148
- fix: added shell function to STRIP by @dylan-conway in https://github.com/oven-sh/bun/pull/1156
- fix compile error by @zhuzilin in https://github.com/oven-sh/bun/pull/1157
- Fix ffi uint64_t parameter by @zhuzilin in https://github.com/oven-sh/bun/pull/1158
- Update WebKit by @Jarred-Sumner in https://github.com/oven-sh/bun/pull/1165
- Support for NULL in ffi by @zhuzilin in https://github.com/oven-sh/bun/pull/1160
- feat: hack in support for tsconfig
extends
by @yepitschunked in https://github.com/oven-sh/bun/pull/1147 - More reliable macOS event loop by @Jarred-Sumner in https://github.com/oven-sh/bun/pull/1166
- chore: Clean buffer C API by @zhuzilin in https://github.com/oven-sh/bun/pull/1174
- Fixed JSBuffer write issues by @nullhook in https://github.com/oven-sh/bun/pull/1175
- Add profiler support by @bwasti in https://github.com/oven-sh/bun/pull/1110
- chore(doc): remove recurse flag from xattr by @jbergstroem in https://github.com/oven-sh/bun/pull/1182
- Fix typo in futex.zig by @eltociear in https://github.com/oven-sh/bun/pull/1186
- Add native StringDecoder by @zhuzilin in https://github.com/oven-sh/bun/pull/1188
- Fix failing Buffer tests by @zhuzilin in https://github.com/oven-sh/bun/pull/1197
- allow set proxy for github by @usrtax in https://github.com/oven-sh/bun/pull/1198
- export syntax error fix for exported enums and namespaces by @dylan-conway in https://github.com/oven-sh/bun/pull/1203
- Plugin API by @Jarred-Sumner in https://github.com/oven-sh/bun/pull/1199
- fix: GitHub CLI installation by @Kit-p in https://github.com/oven-sh/bun/pull/1204
- chore(install-script): automatically add bun to path - bash shell by @xHyroM in https://github.com/oven-sh/bun/pull/1168
- update example react-is to v18(#1155) by @tHyt-lab in https://github.com/oven-sh/bun/pull/1172
- Add native ReadableState by @zhuzilin in https://github.com/oven-sh/bun/pull/1210
New Contributors
- @mljlynch made their first contribution in https://github.com/oven-sh/bun/pull/1124
- @samfundev made their first contribution in https://github.com/oven-sh/bun/pull/1132
- @MNThomson made their first contribution in https://github.com/oven-sh/bun/pull/1148
- @dylan-conway made their first contribution in https://github.com/oven-sh/bun/pull/1156
- @yepitschunked made their first contribution in https://github.com/oven-sh/bun/pull/1147
- @nullhook made their first contribution in https://github.com/oven-sh/bun/pull/1175
- @bwasti made their first contribution in https://github.com/oven-sh/bun/pull/1110
- @jbergstroem made their first contribution in https://github.com/oven-sh/bun/pull/1182
- @usrtax made their first contribution in https://github.com/oven-sh/bun/pull/1198
- @Kit-p made their first contribution in https://github.com/oven-sh/bun/pull/1204
- @tHyt-lab made their first contribution in https://github.com/oven-sh/bun/pull/1172