The bun
CLI contains a Node.js-compatible package manager designed to be a dramatically faster replacement for npm
, yarn
, and pnpm
. It's a standalone tool that will work in pre-existing Node.js projects; if your project has a package.json
, bun install
can help you speed up your workflow.
⚡️ 25x faster — Switch from npm install
to bun install
in any Node.js project to make your installations up to 25x faster.
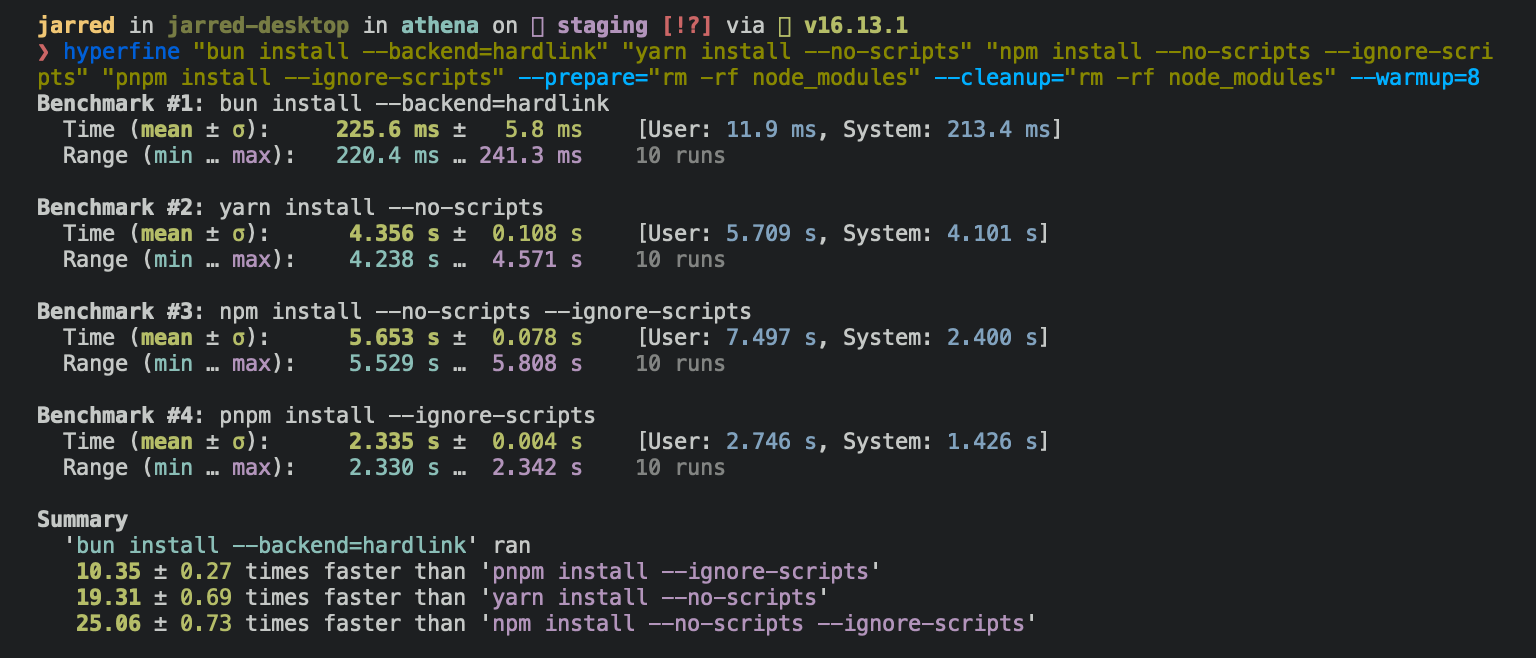
For Linux users
To install all dependencies of a project:
bun install
Running bun install
will:
- Install all
dependencies
,devDependencies
, andoptionalDependencies
. Bun will installpeerDependencies
by default. - Run your project's
{pre|post}install
and{pre|post}prepare
scripts at the appropriate time. For security reasons Bun does not execute lifecycle scripts of installed dependencies. - Write a
bun.lock
lockfile to the project root.
Logging
To modify logging verbosity:
bun install --verbose # debug logging
bun install --silent # no logging
Lifecycle scripts
Unlike other npm clients, Bun does not execute arbitrary lifecycle scripts like postinstall
for installed dependencies. Executing arbitrary scripts represents a potential security risk.
To tell Bun to allow lifecycle scripts for a particular package, add the package to trustedDependencies
in your package.json.
{
"name": "my-app",
"version": "1.0.0",
"trustedDependencies": ["my-trusted-package"]
}
Then re-install the package. Bun will read this field and run lifecycle scripts for my-trusted-package
.
Lifecycle scripts will run in parallel during installation. To adjust the maximum number of concurrent scripts, use the --concurrent-scripts
flag. The default is two times the reported cpu count or GOMAXPROCS.
bun install --concurrent-scripts 5
Workspaces
Bun supports "workspaces"
in package.json. For complete documentation refer to Package manager > Workspaces.
{
"name": "my-app",
"version": "1.0.0",
"workspaces": ["packages/*"],
"dependencies": {
"preact": "^10.5.13"
}
}
Installing dependencies for specific packages
In a monorepo, you can install the dependencies for a subset of packages using the --filter
flag.
# Install dependencies for all workspaces except `pkg-c`
bun install --filter '!pkg-c'
# Install dependencies for only `pkg-a` in `./packages/pkg-a`
bun install --filter './packages/pkg-a'
For more information on filtering with bun install
, refer to Package Manager > Filtering
Overrides and resolutions
Bun supports npm's "overrides"
and Yarn's "resolutions"
in package.json
. These are mechanisms for specifying a version range for metadependencies—the dependencies of your dependencies. Refer to Package manager > Overrides and resolutions for complete documentation.
{
"name": "my-app",
"dependencies": {
"foo": "^2.0.0"
},
"overrides": {
"bar": "~4.4.0"
}
}
Global packages
To install a package globally, use the -g
/--global
flag. Typically this is used for installing command-line tools.
bun install --global cowsay # or `bun install -g cowsay`
cowsay "Bun!"
______
< Bun! >
------
\ ^__^
\ (oo)\_______
(__)\ )\/\
||----w |
|| ||
Production mode
To install in production mode (i.e. without devDependencies
or optionalDependencies
):
bun install --production
For reproducible installs, use --frozen-lockfile
. This will install the exact versions of each package specified in the lockfile. If your package.json
disagrees with bun.lock
, Bun will exit with an error. The lockfile will not be updated.
bun install --frozen-lockfile
For more information on Bun's lockfile bun.lock
, refer to Package manager > Lockfile.
Omitting dependencies
To omit dev, peer, or optional dependencies use the --omit
flag.
# Exclude "devDependencies" from the installation. This will apply to the
# root package and workspaces if they exist. Transitive dependencies will
# not have "devDependencies".
bun install --omit dev
# Install only dependencies from "dependencies"
bun install --omit=dev --omit=peer --omit=optional
Dry run
To perform a dry run (i.e. don't actually install anything):
bun install --dry-run
Non-npm dependencies
Bun supports installing dependencies from Git, GitHub, and local or remotely-hosted tarballs. For complete documentation refer to Package manager > Git, GitHub, and tarball dependencies.
{
"dependencies": {
"dayjs": "git+https://github.com/iamkun/dayjs.git",
"lodash": "git+ssh://github.com/lodash/lodash.git#4.17.21",
"moment": "git@github.com:moment/moment.git",
"zod": "github:colinhacks/zod",
"react": "https://registry.npmjs.org/react/-/react-18.2.0.tgz",
"bun-types": "npm:@types/bun"
}
}
Configuration
The default behavior of bun install
can be configured in bunfig.toml
. The default values are shown below.
[install]
# whether to install optionalDependencies
optional = true
# whether to install devDependencies
dev = true
# whether to install peerDependencies
peer = true
# equivalent to `--production` flag
production = false
# equivalent to `--save-text-lockfile` flag
saveTextLockfile = false
# equivalent to `--frozen-lockfile` flag
frozenLockfile = false
# equivalent to `--dry-run` flag
dryRun = false
# equivalent to `--concurrent-scripts` flag
concurrentScripts = 16 # (cpu count or GOMAXPROCS) x2
CI/CD
Looking to speed up your CI? Use the official oven-sh/setup-bun
action to install bun
in a GitHub Actions pipeline.
name: bun-types
jobs:
build:
name: build-app
runs-on: ubuntu-latest
steps:
- name: Checkout repo
uses: actions/checkout@v4
- name: Install bun
uses: oven-sh/setup-bun@v2
- name: Install dependencies
run: bun install
- name: Build app
run: bun run build